Java Interview Question Part 1
Q. Flow of Memory allocation in Java
A. Two type of memory used to allocate the memory in java:
Q. Garbage collection in Java 7 and Java 8 (JVM Parameter)?
A. Garbage Collection deals with finding and deleting the garbage from memory. It tracks each and every object available in the JVM heap space and removes unused ones.
GC works in two simple steps known as Mark and Sweep:
Q. Type of Garbage Collector and Difference between them in Java?
A. JVM has four types of GC implementations:
A. The adapter design pattern allows two classes of a different interface to work together, without changing any code on either side.
Example: We can use adapter pattern when we work with third-party libraries and we don't use third party library class or interface in our real code directly. We use that time Adapter which works as a bridge between our code and that third party library. Because in the future if we need to change our library then we've to change in the Adapter class only instead of a whole application.
Q. Struts2 Flow
A. The flow of struts 2 application, is combined with many components such as Controller, ActionProxy, ActionMapper, Configuration Manager, ActionInvocation, Interceptor, Action, Result etc.
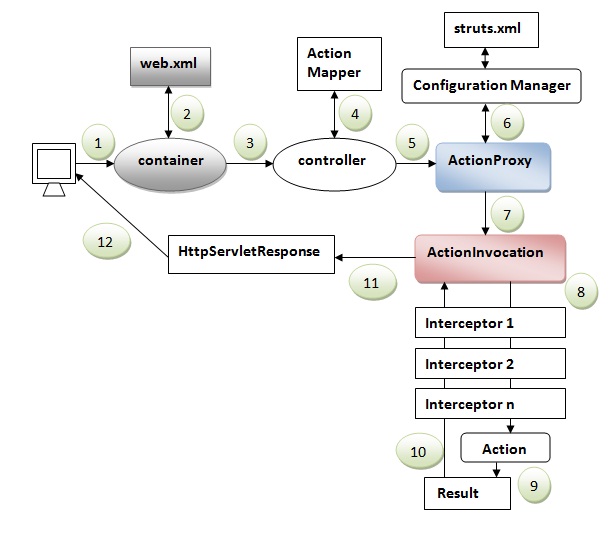
A. Two type of memory used to allocate the memory in java:
- Heap Space
- Stack Space
Java Heap space is used by java runtime to allocate memory to Objects and JRE classes. Whenever we create an object, it’s always created in the Heap space.
Garbage Collection runs on the heap memory to free the memory used by objects that don’t have any reference. Any object created in the heap space has global access.
Java Stack memory is used for execution of a thread. They contain method specific values that are short-lived and references to other objects in the heap that is getting referred from the method.
Stack memory is always referenced in LIFO (Last-In-First-Out) order. Whenever a method is invoked, a new block is created in the stack memory for the method to hold local primitive values and reference to other objects in the method.
Let’s understand the Heap and Stack memory usage with a simple program.
package com.tellmeho.test; public class Memory { public static void main(String[] args) { // Line 1 Java Runtime creates stack memory int i=1; // Line 2 created and stored in the stack memory
Object obj = new Object(); // Line 3 Created in Heap memory &
// Stack memory contains the reference for it Memory mem = new Memory(); // Line 4 Same for above object
mem.foo(obj); // Line 5 a block in the top of the stack is created to be
// used by foo() method } // Line 9 private void foo(Object param) { // Line 6 a new reference to Object is created
// in the foo() stack block
String str = param.toString(); // Line 7 it goes in the String Pool
// in the heap space System.out.println(str); } // Line 8 }

Let’s go through the steps of execution of the program.
- As soon as we run the program, it loads all the Runtime classes into the Heap space. When main() method is found at line 1, Java Runtime creates stack memory to be used by main() method thread.
- We are creating primitive local variable at line 2, so it’s created and stored in the stack memory of main() method.
- Since we are creating an Object in line 3, it’s created in Heap memory and stack memory contains the reference for it. Similar process occurs when we create Memory object in line 4.
- Now when we call foo() method in line 5, a block in the top of the stack is created to be used by foo() method. Since Java is pass by value, a new reference to Object is created in the foo() stack block in line 6.
- A string is created in line 7, it goes in the String Pool in the heap space and a reference is created in the foo() stack space for it.
- foo() method is terminated in line 8, at this time memory block allocated for foo() in stack becomes free.
- In line 9, main() method terminates and the stack memory created for main() method is destroyed. Also the program ends at this line, hence Java Runtime frees all the memory and end the execution of the program.
A. Garbage Collection deals with finding and deleting the garbage from memory. It tracks each and every object available in the JVM heap space and removes unused ones.
GC works in two simple steps known as Mark and Sweep:
- Mark – where the garbage collector identifies which pieces of memory are in use and which are not
- Sweep – removes identified objects during the “mark” phase
It's the role of garbage collection in Java. Garbage collection is run on Heap Memory.
Heap memory has three major areas,
The young generation is the place where all the new objects are created. And, when the young generation is filled, garbage collection is performed. This garbage collection is called Minor GC.
It is divided into three parts – Eden Memory and two Survivor Memory spaces.
Important Points about Young Generation Spaces:
Heap memory has three major areas,
- Young Generation
- Old Generation (instances promoted from S1 to tenured)
- Permanent Generation (contains meta information like class, method detail)

It is divided into three parts – Eden Memory and two Survivor Memory spaces.
Important Points about Young Generation Spaces:
- Whenever new object created, is located in the Eden memory space.
- And when Eden space is filled with objects, Minor GC is performed and all the survivor objects are moved to one of the survivor spaces.
- Minor GC also checks the survivor objects and move them to the other survivor space. That means every time one survivor space would be empty
- When the Objects that are survived after many cycles of GC are moved to the Old generation memory space.
Old Generation memory contains the objects that are long-lived and survived after many rounds of Minor GC. Garbage collection is performed in Old Generation memory when it’s full. And that is called Major GC.
In the Permanent Generation or we can say Permgen stored Class definition, as are static instances. The PermGen is garbage collected like the other parts of the heap.
But the Permanent Generation (Permgen) space is removed from Java 8 features and instead, they introduced MetaSpace.
By this, One of the most common error, “java.lang.OutOfMemoryError: PermGen error” will no longer be seen from Java 8. Because Default MetaSpace is unlimited, it uses system memory as the memory.
Default Garbage collection:
Java 7 - Parallel GC
Java 8 - Parallel GC
Java 9 - G1(Garbage First) GC
Java 10- G1(Garbage First)
GC
Q. Type of Garbage Collector and Difference between them in Java?
A. JVM has four types of GC implementations:
- Serial Garbage Collector (java -XX:+UseSerialGC -jar Application.java)
- Parallel Garbage Collector (-XX:+UseParallelGC)
- CMS (Concurrent Mark Sweep) Garbage Collector (-XX:+UseConcMarkSweepGC)
- G1(Garbage First) Garbage Collector (-XX:+UseG1GC)
Q. Difference between 32-bit and 64-bit JVM?
A. On a 32-bit machine, we have to install 32-bit java/JRE. On a 64-bit machine, we can choose between 32-bit java and 64-bit java both will work fine here. And 64- bit has more memory space than a 32-bit machine. But the High availability of memory has its own problems at runtime.
- 64-bit requires 30-50% more heap than 32-bit. The reason is memory layout of 64-bit architecture.
- And Garbage Collector has to extra cautious work due to more heap memory.
- 64-bits JVMs have the benefit of allocating more memory than the 32-bits ones.
- And 64-bits JVMs use native data types, which are good in terms of performance but on the other hand, also needs larger space.
So overall if we're working on small enterprise project where we don't need to use more space then 32-bit is a good choice for us. And if we don't exactly know the space of our project then 64-bit JVM should be good for us.
A. Yes, a .class file generated using a 32-bit java compiler can be used on 64-bit Java. Java bytecode has nothing to do with 32-bit or 64-bit systems.
Because 64-bit systems, it has a compatibility layer called WoW64 (Windows 32-bit on Windows 64-bit). It helps the processor to switch between 32-bit and 64-bit modes depending on which thread needs to be executed at that time.
Q. Difference between String Buffer Vs String Builder?
A. Both StringBuffer and StringBuilder represents mutable String that means we can add/remove characters, substring without creating new objects.
- And StringBuffer is synchronized which means all method which modifies the internal data of StringBuffer is synchronized like append(), insert() and delete(). And StringBuilder is not synchronized.
- append() use for the concat string at last of given string
- insert() use for add string on given place.
- Because of synchronization StringBuffer is considered thread safe which means multiple threads can call its method without compromising internal data structure but StringBuilder is not synchronized so it is not thread-safe.
Q. Difference between Iterator Vs List Iterator?
- An iterator is used for traversing List and Set both. But ListIterator is used to traverse List only, and we cannot traverse Set using ListIterator.
- And We can traverse in only forward direction using Iterator. But when we use ListIterator, we can traverse a List in both the directions (forward and Backward).
- We cannot obtain indexes while using Iterator. But we can find out indexes at any time while traversing using ListIterator. The methods nextIndex() and previousIndex() are used for this purpose.
- We cannot add the element to the collection while traversing it using Iterator, because it throws ConcurrentModificationException when we try to do it. And in ListIterator, We can add an element at any time while traversing a list.
- We cannot replace the existing element value when using Iterator. In ListIterator we can replace the element using set(E e) method.
Q. Difference between Comparable and Comparator?
A. Comparable and Comparator both are the interfaces and can be used to sort collection elements.
- In Comparator compare two objects need to compare, while Comparable interface compares "this" reference with the given object. So only one object is provided which is then compared to "this" reference.
- And Comparable is found in java.lang package. While A Comparator is part of the java.util package.
- Comparable provides a single sorting sequence. And The Comparator provides multiple sorting sequences.
Q. Design pattern in Spring framework
A. There is a lot of design pattern used in Spring Framework like:
A. There is a lot of design pattern used in Spring Framework like:
Following are the design patterns used in Spring Framework
Design Pattern | Details |
---|---|
MVC Pattern | MVC Design Pattern is a software design that separates the following components of a system or subsystem:
|
Proxy Pattern | Spring uses either JDK proxies (preferred wheneven the proxied target implements at least one interface) or CGLIB proxies (if the target object does not implement any interfaces) to create the proxy for a given target bean. Unless configured to do otherwise, Spring AOP performs run-time weaving Suppose we want to log every method entry and exit. This can be achieved by writing log statements in every method at the start and end. But this will require lot of code work. There are various such tasks like Security which need to be applied across all methods or classes. These are known as cross cutting concerns.AOP addresses the problem of cross-cutting concerns, which would be any kind of code that is repeated in different methods and cannot normally be completely refactored into its own module, like with logging or verification. |
Factory Pattern | This pattern is used by spring to load beans using BeanFactory and Application Context. |
Singleton Pattern | Beans defined in spring config files are singletons by default. A singleton bean in Spring and the singleton pattern are quite different. Singleton pattern says that one and only one instance of a particular class will ever be created per classloader. The scope of a Spring singleton is described as "per container per bean". It is the scope of bean definition to a single object instance per Spring IoC container. The default scope in Spring is Singleton. |
Template method Pattern | The template method design pattern is to define an algorithm as a skeleton of operations and leave the details to be implemented by the child classes. The overall structure and sequence of the algorithm are preserved by the parent class. These are used extensively to deal with boilerplate repeated code |
FrontController Pattern | Front Controller is a controller pattern which provides a centralized controller for managing requests. Each client request must go through and be processed by the Front Controller first, no exceptions. All incoming data is delegated to front controller first. Useful for when your application has multiple entry points which you want to centralize through a single point for standardized processing. Spring implements this design pattern using DispatcherServlet, to dispatch incoming requests to the correct controllers. |
View Helper Pattern | View Helper arranges view components for the user and delegates processing to other business components so the view component doesn't have to contain any processing logic other than logic to present views. Spring makes use of custom JSP tags etc to separate code from presentation in views. |
Prototype Pattern | The Prototype pattern is known as a creational pattern, as it is used to construct objects such that they can be decoupled from their implementing systems. It creates objects based on a template of an existing object through cloning. |
DI/IOC Pattern | Dependency Injection/Inversion of Control design pattern allows us to remove the hard-coded dependencies and make our application loosely coupled, extendable and maintainable. We can implement dependency injection in java to move the dependency resolution from compile-time to runtime. |
Q. What is the Adapter Design Pattern?
Example: We can use adapter pattern when we work with third-party libraries and we don't use third party library class or interface in our real code directly. We use that time Adapter which works as a bridge between our code and that third party library. Because in the future if we need to change our library then we've to change in the Adapter class only instead of a whole application.
Q. Struts2 Flow
A. The flow of struts 2 application, is combined with many components such as Controller, ActionProxy, ActionMapper, Configuration Manager, ActionInvocation, Interceptor, Action, Result etc.
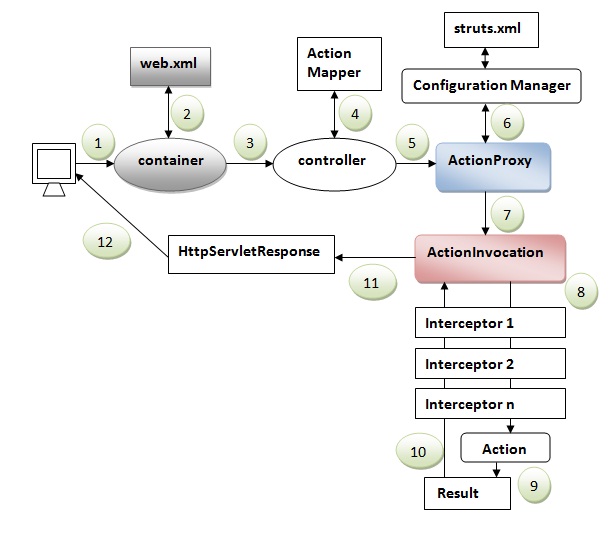
- First of all, a web container will receive a request given by the user.
- Then Web container loads web.xml and verifies whether the URL-patterns are verified or not, if matches web-container transfer the request to FilterDispatcher
- FilterDispatcher handovers the request to ActionProxy, it is a proxy class which is responsible to apply before and after services to original business logic
- ActionProxy contacts ConfiguraionManager class which load the struts.xml file, to know the suitable Action for the request.
- Then request goes back to ActionProxy with the required information.
- ActionPorxy call ActionInvocation which executes the interceptors added to an Action.
- And they receive finally result produced by an action class
- ActionProxy transfers the result back to FilterDispatcher
- And, FilterDispatcher selects an appropriate view, basing on the result
- Finally, FilterDispatcher uses RequestDispatchers forwarding mechanism and forward a view as a response back to the client.
Q. What is Spring Bean Scope?
A. There are five types of spring bean scopes:
- singleton – only one instance of the spring bean will be created for the spring container. This is the default spring bean scope.
- prototype – A new instance will be created every time the bean is requested from the spring container.
- request – This is same as prototype scope, however, it’s meant to be used for web applications. A new instance of the bean will be created for each HTTP request.
- session – A new bean will be created for each HTTP session by the container.
- global-session – This is used to create global session beans for Portlet applications.
Q. Spring MVC flow
- The first request will be received by DispatcherServlet.
- DispatcherServlet will take the help of HandlerMapping and get to know the Controller class name associated with the given request.
- To request a transfer to the Controller, and the controller will process the request by executing appropriate methods and returns ModeAndView object (contains Model data and View name) back to the DispatcherServlet.
- Now DispatcherServlet sends the model object to the ViewResolver to get the actual view page.
- Finally, DispatcherServlet will pass the Model object to the View page to display the result.
Q. Hibernate property (e.g. hibernate dialect)
A. Following is the list of important properties, you will be required to configure for a database in a standalone situation −
Sr.No. | Properties & Description |
---|---|
1 |
hibernate.dialect
This property makes Hibernate generate the appropriate SQL for the chosen database.
Eg.
<property name = "hibernate.dialect"> org.hibernate.dialect.MySQLDialect </property> |
2 |
hibernate.connection.driver_class
The JDBC driver class.
|
3 |
hibernate.connection.url
The JDBC URL to the database instance.
|
4 |
hibernate.connection.username
The database username.
|
5 |
hibernate.connection.password
The database password.
|
6 |
hibernate.connection.pool_size
Limits the number of connections waiting in the Hibernate database connection pool.
|
7 |
hibernate.connection.autocommit
Allows auto-commit mode to be used for the JDBC connection.
|
Q. Hibernate Caching
A. Hibernate caching is used to improves the performance of the application by adding the object to the cache. It is useful when we have to fetch the same data multiple times.
There are mainly two types of caching:
The first level is maintained at the Session level and accessible only to the Session, while the second level cache is maintained at the SessionFactory level and available to all Sessions.
We cannot disable the first level cache, because it always enabled.
Q. Java 8 Features
A. In Java 8, there are many features like:
A lambda expression is an anonymous function. A function that doesn’t have a name and doesn’t belong to any class. To use a lambda expression, we need to use the functional interface. An interface with only a single abstract method is called a functional interface(or Single Abstract method interface), for example, Runnable, callable, ActionListener etc.
Q. What is Serialization and use of byte stream?
A. Serialization is a mechanism to convert an object into a stream of bytes so that it can be written into a file, transported through a network or stored into a database.
Q. What is the difference between HashSet, HashMap and HashTable?
A. Both HashTable and HashMap implements Map interface. While HashSet implements Set.
A. Hibernate caching is used to improves the performance of the application by adding the object to the cache. It is useful when we have to fetch the same data multiple times.
There are mainly two types of caching:
- First level cache (FLC) - by default
- Second level cache (SLC)
The first level is maintained at the Session level and accessible only to the Session, while the second level cache is maintained at the SessionFactory level and available to all Sessions.
We cannot disable the first level cache, because it always enabled.
Q. Java 8 Features
A. In Java 8, there are many features like:
- Lamda Expression:
A lambda expression is an anonymous function. A function that doesn’t have a name and doesn’t belong to any class. To use a lambda expression, we need to use the functional interface. An interface with only a single abstract method is called a functional interface(or Single Abstract method interface), for example, Runnable, callable, ActionListener etc.
- Stream API
- ForEach method
forEach method to iterate over collections and Streams
- String joiner etc.
Using this class we can join more than one strings with the specified delimiter, we can also provide prefix and suffix to that string
Q. What is Serialization and use of byte stream?
A. Serialization is a mechanism to convert an object into a stream of bytes so that it can be written into a file, transported through a network or stored into a database.
In Java there is two type of stream:
Byte Stream and Character Stream
A byte stream access the file byte by byte. This Stream is suitable for any kind of file because Byte oriented streams do not use any encoding scheme while Character oriented streams use character encoding scheme(UNICODE).
Q. What is the difference between HashSet, HashMap and HashTable?
A. Both HashTable and HashMap implements Map interface. While HashSet implements Set.
- Hashtable
Hashtable is basically a data structure to retain values of key-value pair.
- It didn’t allow null for both key and value. You will get NullPointerException if you add null value.
- It is synchronized. So it comes with its cost. Only one thread can access in one time
- HashMap
Like Hashtable it also accepts key-value pair.
- It allows null for both key and value
- It is unsynchronized. So come up with better performance
- HashSet
- HashSet does not allow duplicate values.
- It provides add method rather put method.
- We can use its contain method to check whether the object is already available in HashSet.
- HashSet can be used where you want to maintain a unique list.
Q. What is the Volatile variable in Java?
A. By making a variable volatile using the volatile keyword in Java, we can ensure that its actual value should always be read from main memory and between multiple threads. We can save the cost of synchronization as volatile variables are less expensive than synchronization.
Q. Difference between session.flush and session.clear?
A. flush(): Forces the session to flush. It is used to synchronize session data with a database.
When we call session.flush(), the statements are executed in the database but it will not commit.
If we don’t call session.flush() and if we call session.commit() , internally commit() method executes the statement and commits.
Q. Difference between ConcurrentHashMap and HashTable?
A.
Q. Why use List<Object> list = new ArrayList<Object>(); ?
A.
If we don’t call session.flush() and if we call session.commit() , internally commit() method executes the statement and commits.
Q. Difference between ConcurrentHashMap and HashTable?
A.
- Hashtable comes under the Collection framework and ConcurrentHashMap comes under the Executor framework.
- Hashtable uses a single lock for whole data. ConcurrentHashMap uses multiple locks.
- ConcurrentHashMap doesn't throw a ConcurrentModificationException if one thread tries to modify it while another is iterating over it and does not allow null values.
Q. Why use List<Object> list = new ArrayList<Object>(); ?
A.
List
is an interface,ArrayList
class is a specific implementation of that interface.List<Object> listObject = new ArrayList<Object>();
With this we can change the
List
implementation in future. List listObject
can invoke all the methods declared in the List
interface. In future , if we don't want the ArrayList
implementation of the List
, and change it with say a LinkedList
, we can do that :List<Object> listObject = new LinkedList<Object>();
We will not have to alter the code which uses
listObject
if we had declared the listObject
as List
interface type, and not worry about it breaking the rest of the code because we might have used something specific to ArrayList
with this declaration:ArrayList<Object> listObject = new ArrayList<Object>();
This is called Programming to an interface, not to an implementation.
Q. View Resolver in Spring?
A. View Resolvers are provided by almost all MVC Frameworks, by using View Resolvers models can be rendered in a browser. Spring MVC Framework provides the ViewResolver interface, that maps view names to actual views.
Three important View Resolver implementations provided by Spring MVC, InternalResourceViewResolver, XmlViewResolver and ResourceBundleViewResolver.
In Spring MVC, InternalResourceViewResolver is by default.
Q. Difference between Factory and AbstractFactory Design pattern?
A. Factory
Suppose you are constructing a house and you approach a carpenter to make a door. You give the measurement for the door and your requirements, and he will construct a door for you. In that case, the carpenter is a factory of doors. Your specifications are inputs for the factory, and the door is the output or product from the factory.
Abstract Factory
Now, consider the same example of the door. You can go to a carpenter, or you can go to a plastic door shop. All of them are door factories. Based on the situation, you decide what kind of factory you need to approach. This is like an Abstract Factory.
Q. What is Generics in Java?
A. Generics provide compile-time type checking and removing the risk of
ClassCastException
that was common while working with collection classes.
Q. Difference between ArrayList and LinkedList and Vector?
A. ArrayList and LinkedList both implements List interface and maintains insertion order. Both are non synchronized classes.
ArrayList | LinkedList |
---|---|
1) ArrayList internally uses dynamic array to store the elements. | LinkedList internally uses doubly linked list to store the elements. |
2) Manipulation with ArrayList is slow because it internally uses array. If any element is removed from the array, all the bits are shifted in memory. | Manipulation with LinkedList is faster than ArrayList because it uses doubly linked list so no bit shifting is required in memory. |
3) ArrayList class can act as a list only because it implements List only. | LinkedList class can act as a list and queue both because it implements List and Deque interfaces. |
4) ArrayList is better for storing and accessing data. | LinkedList is better for manipulating data. |
Q. Type of Object Creation in Java?
A. There are five different ways to create an object in Java,
1. Using
new
keyword → constructor get calledEmployee emp1 = new Employee();
2. Using
newInstance()
method of Class
→ constructor get calledEmployee emp2 = (Employee) Class.forName("org.programming.mitra.exercises.Employee")
.newInstance();
It can also be written as
Employee emp2 = Employee.class.newInstance();
3. Using
newInstance()
method of Constructor
→ constructor get calledConstructor<Employee> constructor = Employee.class.getConstructor();
Employee emp3 = constructor.newInstance();
4. Using
clone()
method → no constructor callEmployee emp4 = (Employee) emp3.clone();
5. Using deserialization → no constructor call
ObjectInputStream in = new ObjectInputStream(new FileInputStream("data.obj"));
Employee emp5 = (Employee) in.readObject();
First three methods
new
keyword and both newInstance()
include a constructor call but later two clone and deserialization methods create objects without calling the constructor.
Q. What is Dependency Injection in Spring Framework?
A. Dependency Injection (DI) is a design pattern that removes the dependency from the programming code so that it can be easy to manage and test the application. Dependency Injection makes our programming code loosely coupled.
Spring framework provides two ways to inject dependency
- By Constructor
- By Setter method
Q. Difference between Constructor and Setter Method for Dependency Injection?
A. There are many key differences between constructor injection and setter injection. Setter injection is flexible than Constructor injection.
- Partial dependency: can be injected using setter injection but it is not possible by the constructor. Suppose there are 3 properties in a class, having 3 arg constructor and setters methods. In such case, if you want to pass information for only one property, it is possible by setter method only.
- Overriding: Setter injection overrides the constructor injection. If we use both constructor and setter injection, IOC container will use the setter injection.
- Changes: We can easily change the value by setter injection. It doesn't create a new bean instance always like a constructor. So setter injection is flexible than constructor injection.
Comments
Post a Comment