Java Interview Question Part 2
Q. Different access modifier in java?
A.
Q. Overloading vs Overriding in Java
A.
A.
- A private member is only accessible within the same class as it is declared.
- A member with default (no access modifier) is only accessible within classes in the same package.
- A protected member is accessible within all classes in the same package and within subclasses in other packages.
- A public member is accessible to all classes.
The protected access modifier is accessible within the package and outside the package but through inheritance only.
Q. @Service and @Component
@Service
This annotation is used on a class. The @Service marks a Java class that performs some service, such as execute business logic, perform calculations and call external APIs. This annotation is a specialized form of the@Component annotation intended to be used in the service layer.
@Component
This annotation is used in classes to indicate a Spring component. The @Component annotation marks the Java class as a bean or says component so that the component-scanning mechanism of Spring can add into the application context.
Q. SOAP V/s Rest Web Services
There are many differences between SOAP and REST web services. The important 10 differences between SOAP and REST are given below:
No. | SOAP | REST |
---|---|---|
1) | SOAP is a protocol. | REST is an architectural style. |
2) | SOAP stands for Simple Object Access Protocol. | REST stands for REpresentational State Transfer. |
3) | SOAP can't use REST because it is a protocol. | REST can use SOAP web services because it is a concept and can use any protocol like HTTP, SOAP. |
4) | SOAP uses services interfaces to expose business logic. | REST uses URI to expose business logic. |
5) | JAX-WS is the java API for SOAP web services. | JAX-RS is the java API for RESTful web services. |
6) | SOAP defines standards to be strictly followed. | REST does not define too much standards like SOAP. |
7) | SOAP requires more bandwidth and resource than REST. | REST requires less bandwidth and resource than SOAP. |
8) | SOAP defines its own security. | RESTful web services inherits security measures from the underlying transport. |
9) | SOAP permits XML data format only. | REST permits different data format such as Plain text, HTML, XML, JSON etc. |
10) | SOAP is less preferred than REST. | REST more preferred than SOAP. |
A.
- Overloading happens at compile-time while Overriding happens at runtime: The binding of overloaded method call compile-time and binding of overridden method call Runtime.
- In method overloading, Argument list should be different. And for method Overriding, Argument list should be same.
- In method overloading, Return type of method does not matter, it can be the same or different. While in case of method overriding the method can have a more specific return type.
- Overloading gives better performance compared to overriding. Because the binding of overridden methods is done at runtime.
Q. String vs StringBuffer vs StringBuilder
A. The string is immutable whereas StringBuffer and StringBuilder are mutable classes.
- StringBuffer is threaded safe and synchronized whereas StringBuilder is not, that's why StringBuilder is faster than StringBuffer.
- For String manipulations in the non-multi threaded environment, we should use StringBuilder else use StringBuffer class.
Q. Why String is immutable?
A. String has been mostly used as a parameter for many Java classes e.g. for opening network connection, we can pass hostname and port number as string, we can pass database URL as a string for opening database connection, we can open any file in Java by passing the name of the file as argument to File I/O classes.
In case, if String is not immutable, this would lead serious security threat, I mean someone can access to any file for which he has authorization, and they can change the file name and gain access to that file. Because of immutability, we don't need to worry about that kind of changes or threats on that String object.
Q. Difference between String literal and new keywords?
A. When the String literal used to create String, JVM initially checks weather String with the same value in the String constant pool, if available it creates another reference to it else it creates a new object and stores it in the String constant pool.
In case of a String object, each time we instantiate the class a new object is created given value irrespective of the contents of the String constant pool.
Q. Type of polymorphism
A. There are two types of polymorphism in Java:
- Static Polymorphism also is known as compile time polymorphism or Method Overloading
- Dynamic Polymorphism also is known as runtime polymorphism or Method Overriding.
Q. JDBC Connection in Java
A. JDBC is an acronym for Java Database Connectivity.
There are 5 steps to connect any java application with the database using JDBC. These steps are as follows:
- Register the Driver class (Class.forName())
- Create connection (DriverManager.getConnection( URL, Username, Password ))
- Create statement (con.createStatement())
- Execute queries (stmt.executeQuery( query ))
- Close connection (con.close())
Q. Difference between List and Set
A. List
- Is an Ordered grouping of elements.
- List is used to collection of elements with duplicates.
- Can be accessed by index (ArrayList, LinkedList)
Set
- Is an Unordered grouping of elements.
- HashSet (unordered)
- LinkedHashSet (ordered)
- TreeSet (sorted by natural order or by provided comparator)
- Set is used to collection of elements without duplicates.
- Can not be accessed by index.
Q. Covariant return type
A. The covariant return type in which the return type may vary in the same direction as the subclass.
Suppose : We've class One with get() method whose return type is of One class with return this reference and another class name Two who extends class One with return this reference.
In class Two override the get() method but this time return type would be of class Two with return this reference.
And another method name message with their implementation in class Two.
Now we can call message method in main method by Two().get().message
class One{
One get(){return this;}
}
class Two extends One{
Two get(){return this;}
void message(){
System.out.println("After Java5 welcome to covariant return type");
}
public static void main(String args[]){
new Two().get().message();
}
}
Question on static :
Q. Type of Joins
A. Here are the different types of the JOINs in SQL:
- (INNER) JOIN: Returns records that have matching values in both tables
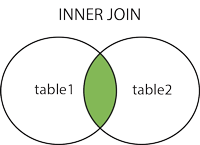
- LEFT (OUTER) JOIN: Return all records from the left table, and the matched records from the right table
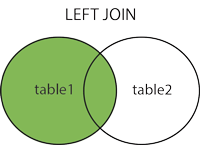
- RIGHT (OUTER) JOIN: Return all records from the right table, and the matched records from the left table
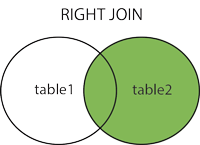
- FULL (OUTER) JOIN: Return all records when there is a match in either left or right table
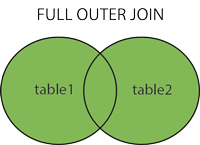
Q. Use of Web.xml
A. The
web.xml
file is the deployment descriptor for a Servlet-based Java web application (which at most Java web apps are). Among other things, it declares which Servlets exist and which URLs they handle.
Q. How HashMap works in Java?
A. HashMap works on hashing principle. In hashing, hash functions are used to link key and value in HashMap.
Objects are stored by calling put(key, value) method of HashMap and retrieved by calling get(key) method.
When we call put method, hashcode() method of the key object is called so that hash function of the map can find a bucket location to store value object, which is actually an index of the internal array, known as the table.
HashMap internally stores mapping in the form of Map. Entry object which contains both key and value object.
And When we want to retrieve the object, we've to call the get() method and again pass the key object. This time again key object generate the same hash code and we end up at same bucket location. If there is only one object then it is returned and that's our value which we add that location.
Q. How HashSet work internally in Java?
A.
HashSet
internally uses HashMap
data structure with a key as generic type E and value as Object
class type.- In HashSet, one static field name PRESENT of
Object type
. Whenever we add any element toHashSet
, it puts an element into backing with theHashMap
key as our element and value as static field PRESENT. - add() method adds the specified element to HashSet if it is not already present and returns true. And, If HashSet already contains the element, returns false.
- add() method internally uses put() method of HashMap.
- put() method returns the previous value associated with key, or null if there was no mapping for key.
- So, if element is not already present in the set, put() method will return null . Hence add() method returns true, because condition in add() method evaluates to true (i.e. null==null)
- If element is already present in the set, put() method will return old value i.e PRESENT which is not null. Hence condition in add() method evaluates to false (i.e. PRESENT == null).
Comments
Post a Comment